前言:虽然现在网上有很多组件库都有轮播图组件,但是我觉得这个东西还是需要自己动手写下好,里面其实有很多有用的知识点的,而且老是用组件库也不好,万一组件库中的轮播图不符合项目的要求或者UI不合适,诸如此类很多情况,还是自己写一个适合自己的最好。
功能分析
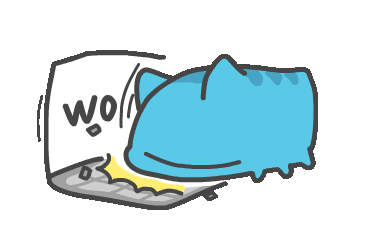
实现思路
HTML中需要包括一个大盒子class=wrap,为轮播图的盒子。一张一张的图片可以用无序列表存储,左右按钮使用button,下方圆点也用无序列表,并为每一个圆点设置计数器data-index。
HTML部分
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <div class="wrap"> <ul class="list"> <li class="item active">0</li> <li class="item">1</li> <li class="item">2</li> <li class="item">3</li> <li class="item">4</li> </ul> <ul class="pointList"> <li class="point active" data-index="0"></li> <li class="point" data-index="1"></li> <li class="point" data-index="2"></li> <li class="point" data-index="3"></li> <li class="point" data-index="4"></li> </ul> <button class="btn" id="leftBtn"></button> <button class="btn" id="rightBtn"></button> </div>
|
CSS部分
- 给wrap盒子一个宽高,list盒子和它同宽同高
- 每一张图片充满盒子,并且都用绝对定位固定在wrap盒子里,让他们有不同的颜色,初始透明度都是0即全透明
- 哪个需要展示,哪个的z-index就变大,并且透明度改为1
- 左右按钮直接使用定位固定在左右两端,小圆点内部使用浮动,再用定位固定在下端
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91
| <style> * { margin: 0; padding: 0; list-style: none; } .wrap{ width: 800px; height: 400px; position: relative; } .list{ width: 800px; height: 400px; position: relative; } .item{ width: 100%; height: 100%; position: absolute; left: 0; opacity: 0; } .item.active { opacity: 1; z-index: 20; } .item:nth-child(1){ background-color: skyblue; } .item:nth-child(2){ background-color: yellowgreen } .item:nth-child(3){ background-color: rebeccapurple; } .item:nth-child(4){ background-color: pink; } .item:nth-child(5){ background-color: orange; } .btn{ width: 50px; height: 100px; position: absolute; top: 50%; z-index: 200; transform: translate(0,-50%); } #leftBtn{ left: 0; } #rightBtn{ right: 0; } .pointList{ height: fit-content; position: absolute; bottom: 20px; right: 20px; z-index: 200; } .point{ width: 10px; height: 10px; background-color: antiquewhite; float: left; margin-right: 8px; border-radius: 100%; border-width: 2px; border-style: solid; border-color: slategray; cursor: pointer; } .point.active { background-color: cadetblue; } </style>
|
Js部分
- 获取元素:包括图片、圆点、按钮、轮播图大盒子
- 需要一个变量index记录当前图片的索引,并且在每次点击的时候要先将样式清空,再根据索引重新赋值(排他思想)
- 点击左右按钮的时候,只需要判断是否为第一张或者最后一张,然后进行+1 -1操作即可
- 点击小圆点时,需要记录点击的圆点的data-index,赋值给Index,然后再执行
- 定义计时器,当鼠标在wrap内,就取消计时,不在wrap内,就开始计时,两秒以后自动播放
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86
| <script> let items = document.querySelectorAll('.item') let points = document.querySelectorAll('.point') let leftBtn = document.querySelector('#leftBtn') let rightBtn = document.querySelector('#rightBtn') let wrap = document.querySelector('.wrap')
var index = 0 var removeActive = function(){ for(var i = 0; i < items.length; i++){ items[i].className = "item" } for(var i = 0; i < points.length; i++){ points[i].className = "point" } } var setActive = function(){ removeActive() items[index].className = "item active" points[index].className = "point active" } var goLeft = function(){ if(index == 0){ index = 4 }else{ index--; } setActive(); } var goRight = function(){ if(index == 4){ index = 0 }else{ index++; } setActive(); } leftBtn.addEventListener('click',function(){ goLeft(); }); rightBtn.addEventListener('click',function(){ goRight(); }) for(var i = 0;i < points.length;i++){ points[i].addEventListener('click',function(){ var pointIndex = this.getAttribute('data-index') index = pointIndex; setActive(); }) } var timer function autoPlay() { timer = setInterval(() => { goRight() }, 2000); } autoPlay() wrap.onmouseover = function(){ clearInterval(timer) console.log('清除计时器') } wrap.onmouseleave = function(){ autoPlay() console.log('加入计时器') }
</script>
|
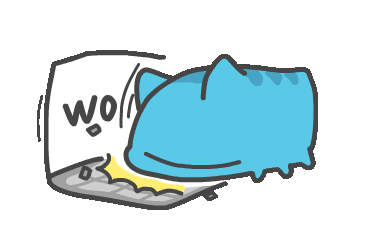
参考:https://www.jb51.net/article/257977.htm